UE4 基于CustomThunk的泛型蓝图节点语法规范 (一)
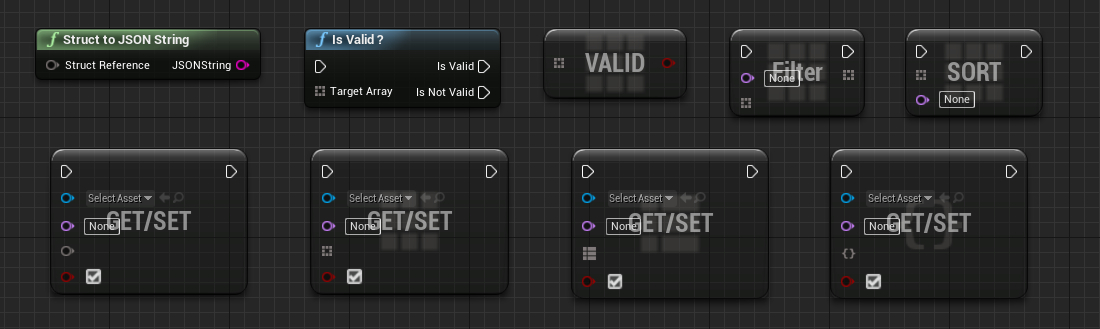
1. Overview
本教程旨在讲解基于CustomThunk方式实现泛型蓝图节点的语法规范,主要包括泛型蓝图节点的组成,泛型蓝图节点的声明,自定义Thunk函数体实现,泛型函数(Generic)的实现案例。因内容较多,故分成二期。如觉有帮助,请不吝点赞;如觉无聊,可一带而过。
2. Introduction
正如前面二期介绍的泛型蓝图节点的应用实例(SORT和GET/SET),泛型蓝图节点应用广泛,并且极大的扩展了蓝图系统的便利性。不同的类型,相同的操作可以使用同一个蓝图节点来完成,减少了代码冗余。本次教程承接上一期内容,继续介绍基于CustomThunk方法的泛型蓝图节点语法规范和实现方式,以下内容为个人分析源码经验所得,如有错漏,敬请谅解,并欢迎在下方评论区中指出,或补充更多相关信息。
CustomThunk和UK2Node都可以实现泛型蓝图节点,二种实现方法大相径庭,实现难易程度也相差很远,具体选择哪一种方法应当考虑一下情况:
1)meta说明符功能可以满足要求时,建议优先使用CustomThunk方法,理由:代码少,debug方便;
- 以下二种情形,建议使用UK2Node方法:
情形一:在编辑模式(Editor)下(非运行状态)需要“修改”蓝图节点引脚(PIN),例如动态增加或删除蓝图节点PIN。
情形二:当使用CustomThunk方式实现的泛型蓝图节点时,程序员对蓝图引脚的控制只有通过meta说明符,而目前meta说明符功能仍存在部分限制。举例,如ue4内置GetDataTableRow蓝图节点一样,该节点的OutRow 引脚(wildcard PIN)需要根据DataTable 引脚输入的DataTable表动态更改OutRow类型;虽然CustomThunk方法也能实现动态改变引脚类型,但是对于此类情况却无法处理。
3. Required Knowledge
-
ue4 c++ 类的创建以及c++中定义蓝图可调用函数;
-
熟悉ue4 c++ UFUNCTION宏中常用说明符以及meta说明符;
4. Approach
4.1 泛型蓝图节点c++函数组成
查看UE4源代码(在整个解决方案中查找关键字CustomThunk),不难发现绝大多数泛型的蓝图节点的c++ 实现包括三个组成部分:(1)带有UFUNCTION宏的函数声明;(2) 自定义Thunk函数体DECLARE_FUNCTION(execFunctionName);(3)真正执行泛型逻辑的Generic_FunctionName()泛型函数。
第(1)部分,限定了在蓝图系统中该函数以蓝图节点方式出现时的样式,包括函数名、参数列表、返回值以及泛型参数等。UFUNCTION宏中包含CustomThunk说明符,其中泛型参数及依赖关系由meta说明符列表决定。
第(2)部分,定义了泛型蓝图函数的Thunk函数体,其实质作用在于运行(Runtime)时获取从蓝图虚拟机VM中传递的参数,其形式规定为 DECLARE_FUNCTION(execFunctionName){}, Function Name为第(1) 部分声明的函数名。
第(3)部分,在完整获得蓝图传递的参数之后,调用蓝图函数的泛型版本,也就是第(3)部分定义的泛型函数。该函数是真正执行泛型功能的代码块,如第2期的数组排序功能,第3期的属性GET/SET功能。此函数命名并无强制性要求,可以选择任意合法的函数名,为了便于理解,源代码中一般采用Generic_(Generic)+ FunctionName的形式,Generic的含义就是通常所说的泛型。
4.2 泛型蓝图函数声明
如蓝图系统中的变量类型(Variable Type)一样,标识泛型函数通配符(wildcard)参数的说明符也有四种,分别为标识单个变量SingleVariable wildcard类型的"CustomStructureParam"说明符、标识容器Array的"ArrayParm"说明符、标识容器Map的"MapParam"说明符、标识容器Set的"SetParam"说明符以及各种辅助说明符,四种说明符存在部分差异。
以下代码编译运行环境:UE4 v4.25 / vs2017
(1)Single Variable泛型参数的函数声明示例
public: // Wildcard property
// Declare a function with an wildcard parameter.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (CustomStructureParam = "Value"), Category = "Utilities|Variadic")
static void PropertyFunction1(const int32& Value);
DECLARE_FUNCTION(execPropertyFunction1) {}
// The variable marked by "CustomStructureParam" would be a placeholder.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (CustomStructureParam = "Value"), Category = "Utilities|Variadic")
static void PropertyFunction2(UProperty* Value);
DECLARE_FUNCTION(execPropertyFunction2) {}
// Declare a function with multiple wildcard parameters.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (CustomStructureParam = "Value1,Value2,Value"), Category = "Utilities|Variadic")
static void PropertyFunction3(const int32& Value1, const int32& Value2, int32& Value);
DECLARE_FUNCTION(execPropertyFunction3) {}
// Declare a function with incorrect delimiter ---> Bad case <---
UFUNCTION(BlueprintCallable, CustomThunk, meta = (CustomStructureParam = "Value1|Value2"), Category = "Utilities|Variadic")
static void PropertyFunction4(const int32& Value1, const int32& Value2);
DECLARE_FUNCTION(execPropertyFunction4) {}
// Declare a function with one incorrect type ---> Bad case <---
UFUNCTION(BlueprintCallable, CustomThunk, meta = (CustomStructureParam = "Value"), Category = "Utilities|Variadic")
static void PropertyFunction5(const TArray<int32>& Value);
DECLARE_FUNCTION(execPropertyFunction5) {}
将以上代码拷贝到以UBlueprintFunctionLibrary为基类的C++类的头文件中(.h文件),编译后即可在蓝图系统中找到这5个蓝图节点。

Single Variable泛型参数的函数声明规则 :
(1) UFUNTIION()宏应包含三类说明符:①表示蓝图可调用含义的说明符,如常用的BlueprintCallable、BlueprintPure;② CustomThunk说明符,标识该函数需要自定义Thunk函数体;③被CustomStructureParam说明符所标识的泛型参数列表,其他说明符视情形选择。(见示例PropertyFunction1 / PropertyFunction2 / PropertyFunction5)
(2) 被CustomStructureParam说明符标识的函数参数实为一个占位符,源代码中常用const int32& VariableName表示一个输入类型泛型参数,int32& VariableName表示一个输出类型的泛型参数。(见示例 PropertyFunction3)
(3) CustomStructureParam说明符标识多个泛型变量时,变量之间需以英文逗号”,”作为分隔符,不得包含其他字符,如空格 、”|”等其他字符;否则虽然可以通过vs编译,但是在蓝图系统不会如期显示。(见示例 PropertyFunction4)
(4) 限制:目前,在源代码中并没有存在(或者说没发现)标识多个Single Variable泛型参数之间依赖关系的辅助说明符,也就是不能定义多个Single Variable 泛型参数彼此之间的依赖关系。
(2)Array泛型参数的蓝图函数声明示例
public: // Wildcard TArray
// Declare a function with one wildcard array parameter.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (ArrayParm = "Array"), Category = "Utilities|Variadic")
static void ArrayFunction1(const TArray<int32>& Array);
DECLARE_FUNCTION(execArrayFunction1) {}
// Declare a function with one wildcard array parameter with an type dependent parameter .
UFUNCTION(BlueprintCallable, CustomThunk, meta = (ArrayParm = "Array", ArrayTypeDependentParams = "Item"), Category = "Utilities|Variadic")
static void ArrayFunction2(const TArray<int32>& Array, const int32& Item);
DECLARE_FUNCTION(execArrayFunction2) {}
// Declare a function with multiple wildcard array parameters.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (ArrayParm = "Array1,Array2,Array3", ArrayTypeDependentParams = "Array1,Array2,Array3"), Category = "Utilities|Variadic")
static void ArrayFunction3(const TArray<int32>& Array1, const TArray<int32>& Array2, TArray<int32>& Array3);
DECLARE_FUNCTION(execArrayFunction3) {}
// Declare a function with multiple wildcard array parameters
UFUNCTION(BlueprintCallable, CustomThunk, meta = (ArrayParm = "Array1,Array2,Array3", ArrayTypeDependentParams = "Array1,Array3"), Category = "Utilities|Variadic")
static void ArrayFunction4(const TArray<int32>& Array1, const TArray<int32>& Array2, TArray<int32>& Array3);
DECLARE_FUNCTION(execArrayFunction4) {}
// Declare a function with multiple wildcard array parameters. ---> Bad case <---
UFUNCTION(BlueprintCallable, CustomThunk, meta = (ArrayParm = "Array1|Array2|Array3"), Category = "Utilities|Variadic")
static void ArrayFunction5(const TArray<int32>& Array1, const TArray<int32>& Array2, TArray<int32>& Array3);
DECLARE_FUNCTION(execArrayFunction5) {}

Wildcard Array泛型函数声明规则 :
(1) UFUNTIION()宏应包含三类说明符:①表示蓝图可调用含义的说明符,如常用的BlueprintCallable、BlueprintPure;② CustomThunk说明符,标识该函数需要自定义Thunk函数体;③被说明符ArrayParm所标识的泛型参数(wildcard array)列表,此外还可能包含标识泛型Array参数之间依赖关系的ArrayTypeDependentParams说明符,其他说明符视情形选择。(见示例 ArrayFunction1 / ArrayFunction2 / ArrayFunction3 / ArrayFunction4)
(2) 源代码中常用const TArray
(3) ArrayParm说明符标识多个泛型参数变量时,变量之间需以英文逗号”,”分隔,不得包含其他字符,否则虽然可以通过编译,但是在蓝图系统中显示异常。(见示例ArrayFunction5)
(4) 被ArrayTypeDependentParams说明符标识的多个泛型Array参数的类型相互依赖,在蓝图图表中被调用时只有确定其中之一,余下泛型Array参数随之变化。 更多泛型Array示例见源码:KismetArrayLibrary.h
(3)Map泛型参数的函数声明示例
public: // wildcard TMap
// Declare a function with one wildcard array parameter.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (MapParam = "TargetMap"), Category = "Utilities|Variadic")
static void MapFunciton1(const TMap<int32, int32>& TargetMap);
DECLARE_FUNCTION(execMapFunciton1) {}
// Declare a function with one wildcard map parameter with type dependent parameters.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (MapParam = "TargetMap", MapKeyParam = "Key", MapValueParam = "Value", AutoCreateRefTerm = "Key, Value"), Category = "Utilities|Variadic")
static void MapFunciton2(const TMap<int32, int32>& TargetMap, const int32& Key, const int32& Value);
DECLARE_FUNCTION(execMapFunciton2) {}
//UNSUPPORTED ---> Declare a function with multiple wildcard map parameters.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (MapParam = "TargetMap,SourceMap,ReturnMap"), Category = "Utilities|Variadic")
static void MapFunciton3(const TMap<int32, int32>& TargetMap, const TMap<int32, int32>& SourceMap, TMap<int32, int32>& ReturnMap);
DECLARE_FUNCTION(execMapFunciton3) {}
//UNSUPPORTED ---> Declare a function with multiple wildcard map parameters.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (MapParam = "TargetMap|SourceMap|ReturnMap"), Category = "Utilities|Variadic")
static void MapFunciton4(const TMap<int32, int32>& TargetMap, const TMap<int32, int32>& SourceMap, TMap<int32, int32>& ReturnMap);
DECLARE_FUNCTION(execMapFunciton4) {}

Wildcard Map泛型函数声明规则 :
(1) UFUNTIION()宏应包含三类说明符:①表示蓝图可调用含义的说明符,如常用的BlueprintCallable、BlueprintPure;② CustomThunk说明符,标识该函数需要自定义Thunk函数体;③被说明符MapParam所标识的泛型Map参数(wildcard map)列表,其他说明符视情形选择。(见示例 MapFunciton1 / MapFunciton2)
(2) 源代码中常用const TMap
(3) 经试验,声明多个泛型Map参数时无论是采用”,”还是”|”分隔变量,在蓝图系统均出现不同情形的异常情况;以逗号分隔多个泛型Map参数时,蓝图西并没有将这些参数标识为泛型Map引脚。”|”分隔多个参数时,虽然该蓝图节点显示为多个泛型Map引脚,但是当连接上Map类型变量时该引脚并没有更新类型,如上图示例MapFunciton3和 MapFunciton4。不确定这是不是一个引擎bug!?
(4) 被MapKeyParam和MapValueParam说明符标识的泛型参数与被MapParam说明符标识的泛型参数相互依赖。 Map没有类似于” ArrayTypeDependentParams”说明符一样标识多个泛型Map参数依赖关系的辅助说明符” MapTypeDependentParams”,(见示例MapFunciton2)。更多泛型Map示例见源码:BlueprintMapLibrary.h。
(4)Set泛型参数的函数声明示例
public:// wildcard Set
// Declare a function with one wildcard Set parameter.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (SetParam = "TargetSet"), Category = "Utilities|Variadic")
static void SetFunciton1(const TSet<int32>& TargetSet);
DECLARE_FUNCTION(execSetFunciton1) {}
// Declare a function with one wildcard Set parameter with type independent parameter.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (SetParam = "TargetSet,NewItem"), Category = "Utilities|Variadic")
static void SetFunciton2(const TSet<int32>& TargetSet, const int32& NewItem);
DECLARE_FUNCTION(execSetFunciton2) {}
// Declare a function with one wildcard Set parameter with type dependent parameter.
UFUNCTION(BlueprintCallable, CustomThunk, meta = (SetParam = "TargetSet|NewItem"), Category = "Utilities|Variadic")
static void SetFunciton3(const TSet<int32>& TargetSet, const int32& NewItem);
DECLARE_FUNCTION(execSetFunciton3) {}
// Declare a function with one wildcard Set parameter and type dependent array parameter
UFUNCTION(BlueprintCallable, CustomThunk, meta = (SetParam = "TargetSet|NewItems"), Category = "Utilities|Variadic")
static void SetFunciton4(const TSet<int32>& TargetSet, const TArray<int32>& NewItems);
DECLARE_FUNCTION(execSetFunciton4) {}
// Declare a function with multiple wildcard Set parameters v1
UFUNCTION(BlueprintCallable, CustomThunk, meta = (SetParam = "Set1|Set2,Set3"), Category = "Utilities|Variadic")
static void SetFunciton5(const TSet<int32>& Set1, const TSet<int32>& Set2, TSet<int32>& Set3);
DECLARE_FUNCTION(execSetFunciton5) {}
// Declare a function with multiple wildcard Set parameters v2
UFUNCTION(BlueprintCallable, CustomThunk, meta = (SetParam = "Set1|Set2|Set3"), Category = "Utilities|Variadic")
static void SetFunciton6(const TSet<int32>& Set1, const TSet<int32>& Set2, TSet<int32>& Set3);
DECLARE_FUNCTION(execSetFunciton6) {}

声明规则 :
(1) UFUNTIION()宏应包含三类说明符:①表示蓝图可调用含义的说明符,如常用的BlueprintCallable、BlueprintPure;② CustomThunk说明符,标识该函数需要自定义Thunk函数体;③被说明符SetParam所标识的泛型Set参数(wildcard set)列表,其他说明符视情形选择,(见示例SetFunciton1 / SetFunciton2 / SetFunciton3)。
(2) 源代码中常用const TSet
(3) 声明多个泛型Set参数时, SetParam说明符列表的泛型参数之间可以使用”,”和”|”二种分隔符,(见示例SetFunciton2 / SetFunciton3);SetParam说明符同时也可以标识有依赖关系的泛型Arrary参数,(见示例SetFunciton4)。
(4) 多个泛型参数的依赖关系由分隔符的类型决定,以逗号”,”分隔的多个泛型Set参数之间相互独立,以”|”分隔的多个泛型Set参数之间相互依赖,(见示例SetFunciton5 / SetFunciton6)。更多泛型Set示例见源码:BlueprintSetLibrary.h
4.3 Thunk函数体的实现过程
如前所述,在UFUNCTION宏中使用了CustomThunk说明符,需要为该函数自定义Thunk函数体。Thunk函数体主要作用:当蓝图节点被调用时,获取从蓝图虚拟机VM中传递来的参数值,并将值传递给执行特定功能的c++函数。
首先了解下Thunk函数体的由来。通常定义在c++类中,并用UFUNCTION宏标识为蓝图可调用的函数;编译时,UHT会在该类的generated.h文件中为其自动生成一个形如DECLARE_FUNCTION(execFunctionName) {}的函数体,也就是我们所说的Thunk函数体。
举例来说,在BlueprintFunctionLibrary中声明如下函数
UFUNCTION(BlueprintPure, BlueprintCallable, Category = "Math|Random", meta = (DisplayName = "Random Character", Keywords = "random, char,string"))
static FString GetRandomCharacter(int32 MinValue = 33, int32 MaxValue = 126);
generated.h文件中就存在如下代码块
DECLARE_FUNCTION(execGetRandomCharacter) \
{ \
P_GET_PROPERTY(UIntProperty, Z_Param_MinValue); \
P_GET_PROPERTY(UIntProperty, Z_Param_MaxValue); \
P_FINISH; \
P_NATIVE_BEGIN; \
*(FString*)Z_Param__Result = URandomAlgorithm::GetRandomCharacter(Z_Param_MinValue, Z_Param_MaxValue); \
P_NATIVE_END; \
}

Thunk函数体有其特定的语法规则,要想自定义泛型蓝图节点,就必然要学会编写Thunk函数体。熟练掌握Thunk函数体的语法规则,就可以随心所欲的自定义Thunk函数体。Thunk函数体的用途在于Runtime时获取蓝图VM传递过来的参数值,因而Thunk函数的代码是在Runtime时运行的。在自定义Thunk函数体中所使用的宏定义于UObject\ScriptMacros.h文件中。
自定义Thunk函数体包含如下规则:
(1)Thunk函数的基本形式如下, DECLARE_FUNCTION(execFunctionName) {},FucntionName为函数声明时的函数名称。在花括号{}内部包含的代码:P_FINISH宏之前为获取函数参数的代码块、P_NATIVE_BEGIN和P_NATIVE_END宏包裹着真正执行该函数功能的被调用函数,若是泛型蓝图节点,此处调用该函数的泛型版本(泛型函数)。若函数无返回值,可以直接调用泛型函数;若存在返回值,则应当在"="左侧定义适当的返回值类型。
DECLARE_FUNCTION(execFunctionName)
{
// Get Parameters
P_FINISH;
P_NATIVE_BEGIN;
*(FString*)Result = Generic_FunctionName(); // Call generic function
P_NATIVE_END;
}
(2)当在Thunk函数体中获取多个参数时,获取的先后次序应与函数声明时变量在参数列表中出现的次序保持一致,否则在函数被调用时将引发程序崩溃。
(3)在Thunk函数体中,泛型蓝图函数的参数列表中确定类型的参数(如bool / uint8 / int32 / float / FName / FString等)和泛型参数(wilcard SingleVariable / TArray / TMap / TSet)获取方式不同。
a.获取确定类型的函数参数的代码的编写方法:
如下示例,可以先定义一个带该已知类型的函数,编译后在该类.generated.h文件中找到同名的Thunk体后,并定位到获取该类型参数的代码块。按同样的规则,在自定义的Thunk函数体中,编写获取该参数的代码即可,这种方式对于新手非常有用。
确定类型变量函数声明示例:
UFUNCTION(BlueprintCallable, Category = "MyProject")
static void TestFunction(
bool BoolVar
, uint8 ByteVar
, int32 IntegerVar
, float FloatVar
, FName NameVar
, FString StringVar
, const FText& TextVar
, FVector VectorVar
, FTransform TransformVar
, UObject* ObjectVar
, TSubclassOf<UObject> ClassVar
, bool& RetBoolVar
, uint8& RetByteVar
, int32& RetIntegerVar
, float& RetFloatVar
, FName& RetNameVar
, FString& RetStringVar
, FText& RetTextVar
, FVector& RetVectorVar
, FTransform& RetTransformVar
, UObject*& RetObjectVar
, TSubclassOf<UObject>& RetClassVar
) ;
对应的自动生成的Thunk函数体示例:
DECLARE_FUNCTION(execTestFunction) \
{ \
P_GET_UBOOL(Z_Param_BoolVar); \
P_GET_PROPERTY(UByteProperty, Z_Param_ByteVar); \
P_GET_PROPERTY(UIntProperty, Z_Param_IntegerVar); \
P_GET_PROPERTY(UFloatProperty, Z_Param_FloatVar); \
P_GET_PROPERTY(UNameProperty, Z_Param_NameVar); \
P_GET_PROPERTY(UStrProperty, Z_Param_StringVar); \
P_GET_PROPERTY_REF(UTextProperty, Z_Param_Out_TextVar); \
P_GET_STRUCT(FVector, Z_Param_VectorVar); \
P_GET_STRUCT(FTransform, Z_Param_TransformVar); \
P_GET_OBJECT(UObject, Z_Param_ObjectVar); \
P_GET_OBJECT(UClass, Z_Param_ClassVar); \
P_GET_UBOOL_REF(Z_Param_Out_RetBoolVar); \
P_GET_PROPERTY_REF(UByteProperty, Z_Param_Out_RetByteVar); \
P_GET_PROPERTY_REF(UIntProperty, Z_Param_Out_RetIntegerVar); \
P_GET_PROPERTY_REF(UFloatProperty, Z_Param_Out_RetFloatVar); \
P_GET_PROPERTY_REF(UNameProperty, Z_Param_Out_RetNameVar); \
P_GET_PROPERTY_REF(UStrProperty, Z_Param_Out_RetStringVar); \
P_GET_PROPERTY_REF(UTextProperty, Z_Param_Out_RetTextVar); \
P_GET_STRUCT_REF(FVector, Z_Param_Out_RetVectorVar); \
P_GET_STRUCT_REF(FTransform, Z_Param_Out_RetTransformVar); \
P_GET_OBJECT_REF(UObject, Z_Param_Out_RetObjectVar); \
P_GET_OBJECT_REF_NO_PTR(TSubclassOf<UObject>, Z_Param_Out_RetClassVar); \
P_FINISH; \
P_NATIVE_BEGIN; \
UMiscAlgorithm::TestFunction(Z_Param_BoolVar, Z_Param_ByteVar, Z_Param_IntegerVar, Z_Param_FloatVar, Z_Param_NameVar, Z_Param_StringVar, Z_Param_Out_TextVar, Z_Param_VectorVar, Z_Param_TransformVar, Z_Param_ObjectVar, Z_Param_ClassVar, Z_Param_Out_RetBoolVar, Z_Param_Out_RetByteVar, Z_Param_Out_RetIntegerVar, Z_Param_Out_RetFloatVar, Z_Param_Out_RetNameVar, Z_Param_Out_RetStringVar, Z_Param_Out_RetTextVar, Z_Param_Out_RetVectorVar, Z_Param_Out_RetTransformVar, Z_Param_Out_RetObjectVar, Z_Param_Out_RetClassVar); \
P_NATIVE_END; \
} \
b.获取泛型参数的代码的编写方法:
ue4 v4.25以上版本 UProperty已被FProperty替代,本质没变,只需稍作修改。以下代码为4.25之前版本。获取泛型参数需要同时获取该变量地址void和变量属性UProperty/ UArrayProperty* / UMapProperty* / USetProperty*,如下示例:
获取泛型Single Variable
Stack.StepCompiledIn<UStructProperty>(NULL);
void* SrcPropertyAddr = Stack.MostRecentPropertyAddress;
UProperty* SrcProperty = Cast<UProperty>(Stack.MostRecentProperty);
获取泛型Array Variable
Stack.StepCompiledIn<UArrayProperty>(NULL);
void* SrcArrayAddr = Stack.MostRecentPropertyAddress;
UArrayProperty* SrcArrayProperty = Cast<UArrayProperty>(Stack.MostRecentProperty);
获取泛型Map Variable
Stack.MostRecentProperty = nullptr;
Stack.StepCompiledIn<UMapProperty>(NULL);
void* SrcMapAddr = Stack.MostRecentPropertyAddress;
UMapProperty* SrcMapProperty = Cast<UMapProperty>(Stack.MostRecentProperty);
获取泛型Set Variable
Stack.MostRecentProperty = nullptr;
Stack.StepCompiledIn<USetProperty>(NULL);
void* SetAddr = Stack.MostRecentPropertyAddress;
USetProperty* SetProperty = Cast<USetProperty>(Stack.MostRecentProperty);
5. Conclusion
以上内容讲述了泛型蓝图节点的三个组成部分的主要功能,以及带泛型参数蓝图节点的声明方法和自定义Thunk函数体的实现过程。为避免文章过长,在下一节 UE4 基于CustomThunk的泛型蓝图节点语法规范 (二), 将分享几个自己编写的泛型蓝图节点**,**让大家对自定义泛型蓝图节点的第三部分-泛型函数(Generic)有加全面而清晰认识。